The Forge
Action
An action is what a block can do when it is executed with Typebot. A block can have multiple actions.
Here is the sendMessage
action of the Telegram block:
import { createAction, option } from '@typebot.io/forge'
import { auth } from '../auth'
import ky from 'ky'
export const sendMessage = createAction({
auth,
name: 'Send message',
options: option.object({
chatId: option.string.layout({
label: 'Chat ID',
placeholder: '@username',
}),
text: option.string.layout({
label: 'Message text',
input: 'textarea',
}),
}),
run: {
server: async ({ credentials: { token }, options: { chatId, text } }) => {
try {
await ky.post(`https://api.telegram.org/bot${token}/sendMessage`, {
json: {
chat_id: chatId,
text,
},
})
} catch (error) {
console.log('ERROR', await error.response.text())
}
},
},
})
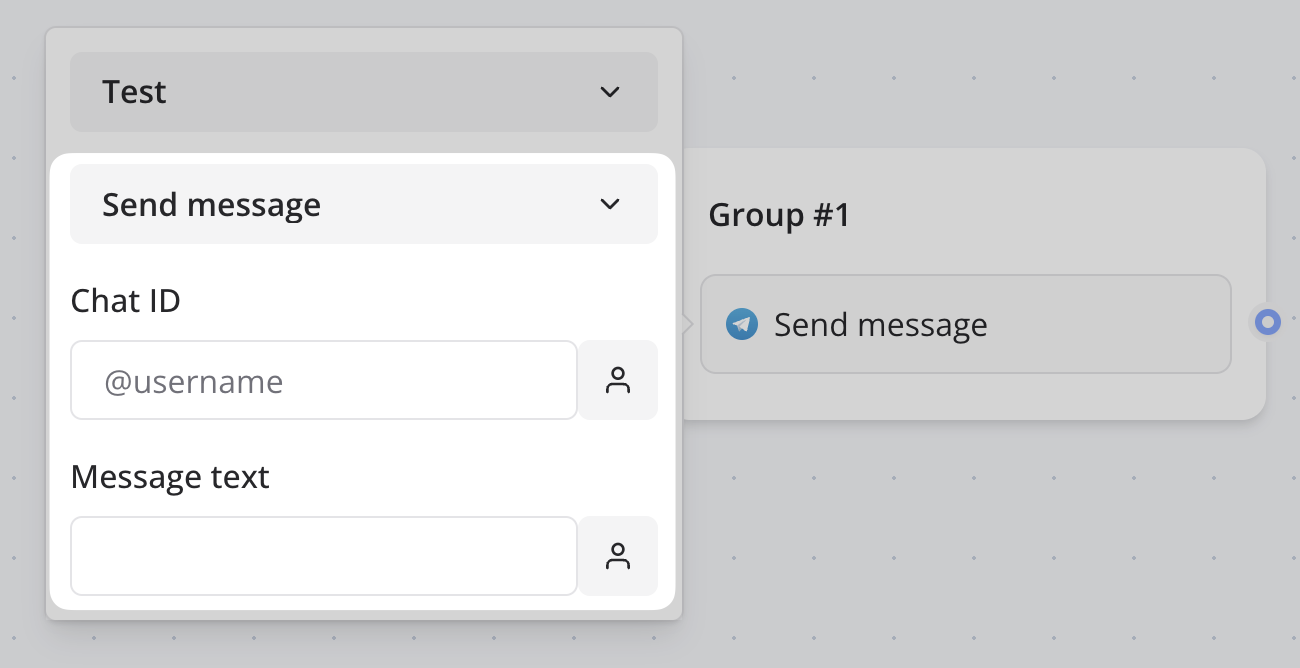
Props
name
string
requiredThe name of the action.
auth
Auth
If the block requires authentication, the auth object needs to be passed to the action.
baseOptions
z.ZodObject<any>
If the block has options defined (see block props), this needs to be provided here.
options
z.ZodObject<any>
The action configuration options. See Options for more information.
run
Check out the Run documentation for more information on how this can be configured depending of your scenario.